Mesa le prend en charge. Si vous souhaitez vous limiter à OpenGL ES uniquement vous devrez alors le créer dans un répertoire séparé, puis ajouter les répertoires d'inclusion et de bibliothèque appropriés.
Mise à jour :
Vous pouvez (toujours) utiliser le SDK PowerVR et maintenant il prend également en charge Vulkan. Liens mis à jour :
- Page SDK PowerVR :https://www.imgtec.com/developers/powervr-sdk-tools/powervr-sdk/
- Page de téléchargement des programmes d'installation :https://www.imgtec.com/developers/powervr-sdk-tools/installers/
- Dépôt Github :https://github.com/powervr-graphics/Native_SDK
Au moment de ma réponse initiale, PowerVR SDK était la solution la plus complète (Mesa a obtenu une prise en charge complète d'OpenGL ES 2.0 avec sa version 3.1 selon sa page Wikipedia).
Désormais, Mesa et Mali SDK peuvent également être un choix. Pour des informations détaillées sur ceux-ci, veuillez vous référer à cette réponse détaillée de Ciro Santilli 冠状病毒审查六四事件法轮功
Réponse originale :
Vous pouvez utiliser POWERVR SDK pour émuler Opengl es sur votre PC. Vous pouvez télécharger le SDK ici. L'archive fournit les étapes nécessaires pour installer les bibliothèques d'émulation sous forme de fichier de documentation et comprend des didacticiels et des applications de démonstration avec les codes sources.
GLFW, Mesa, Ubuntu 16.04 AMD64
Ce n'était pas facile à configurer sur Ubuntu 14.04, mais maintenant ça marche.
sudo apt-get install libglfw3-dev libgles2-mesa-dev
gcc glfw_triangle.c -lGLESv2 -lglfw
Sortie :

glfw_triangle.c
#include <stdio.h>
#include <stdlib.h>
#define GLFW_INCLUDE_ES2
#include <GLFW/glfw3.h>
static const GLuint WIDTH = 800;
static const GLuint HEIGHT = 600;
static const GLchar* vertex_shader_source =
"#version 100\n"
"attribute vec3 position;\n"
"void main() {\n"
" gl_Position = vec4(position, 1.0);\n"
"}\n";
static const GLchar* fragment_shader_source =
"#version 100\n"
"void main() {\n"
" gl_FragColor = vec4(1.0, 0.0, 0.0, 1.0);\n"
"}\n";
static const GLfloat vertices[] = {
0.0f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f,
};
GLint common_get_shader_program(const char *vertex_shader_source, const char *fragment_shader_source) {
enum Consts {INFOLOG_LEN = 512};
GLchar infoLog[INFOLOG_LEN];
GLint fragment_shader;
GLint shader_program;
GLint success;
GLint vertex_shader;
/* Vertex shader */
vertex_shader = glCreateShader(GL_VERTEX_SHADER);
glShaderSource(vertex_shader, 1, &vertex_shader_source, NULL);
glCompileShader(vertex_shader);
glGetShaderiv(vertex_shader, GL_COMPILE_STATUS, &success);
if (!success) {
glGetShaderInfoLog(vertex_shader, INFOLOG_LEN, NULL, infoLog);
printf("ERROR::SHADER::VERTEX::COMPILATION_FAILED\n%s\n", infoLog);
}
/* Fragment shader */
fragment_shader = glCreateShader(GL_FRAGMENT_SHADER);
glShaderSource(fragment_shader, 1, &fragment_shader_source, NULL);
glCompileShader(fragment_shader);
glGetShaderiv(fragment_shader, GL_COMPILE_STATUS, &success);
if (!success) {
glGetShaderInfoLog(fragment_shader, INFOLOG_LEN, NULL, infoLog);
printf("ERROR::SHADER::FRAGMENT::COMPILATION_FAILED\n%s\n", infoLog);
}
/* Link shaders */
shader_program = glCreateProgram();
glAttachShader(shader_program, vertex_shader);
glAttachShader(shader_program, fragment_shader);
glLinkProgram(shader_program);
glGetProgramiv(shader_program, GL_LINK_STATUS, &success);
if (!success) {
glGetProgramInfoLog(shader_program, INFOLOG_LEN, NULL, infoLog);
printf("ERROR::SHADER::PROGRAM::LINKING_FAILED\n%s\n", infoLog);
}
glDeleteShader(vertex_shader);
glDeleteShader(fragment_shader);
return shader_program;
}
int main(void) {
GLuint shader_program, vbo;
GLint pos;
GLFWwindow* window;
glfwInit();
glfwWindowHint(GLFW_CLIENT_API, GLFW_OPENGL_ES_API);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 2);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 0);
window = glfwCreateWindow(WIDTH, HEIGHT, __FILE__, NULL, NULL);
glfwMakeContextCurrent(window);
printf("GL_VERSION : %s\n", glGetString(GL_VERSION) );
printf("GL_RENDERER : %s\n", glGetString(GL_RENDERER) );
shader_program = common_get_shader_program(vertex_shader_source, fragment_shader_source);
pos = glGetAttribLocation(shader_program, "position");
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
glViewport(0, 0, WIDTH, HEIGHT);
glGenBuffers(1, &vbo);
glBindBuffer(GL_ARRAY_BUFFER, vbo);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(pos, 3, GL_FLOAT, GL_FALSE, 0, (GLvoid*)0);
glEnableVertexAttribArray(pos);
glBindBuffer(GL_ARRAY_BUFFER, 0);
while (!glfwWindowShouldClose(window)) {
glfwPollEvents();
glClear(GL_COLOR_BUFFER_BIT);
glUseProgram(shader_program);
glDrawArrays(GL_TRIANGLES, 0, 3);
glfwSwapBuffers(window);
}
glDeleteBuffers(1, &vbo);
glfwTerminate();
return EXIT_SUCCESS;
}
Les principales lignes de code sont :
#define GLFW_INCLUDE_ES2
#include <GLFW/glfw3.h>
GLFW_INCLUDE_ES2
est documenté sur :http://www.glfw.org/docs/latest/build_guide.html#build_macros et un rapide coup d'œil à la source montre qu'il est transmis à GLES :
#elif defined(GLFW_INCLUDE_ES2)
#include <GLES2/gl2.h>
#if defined(GLFW_INCLUDE_GLEXT)
#include <GLES2/gl2ext.h>
#endif
Cette source semble être écrite dans le sous-ensemble commun de GLES et OpenGL (comme une grande partie de GLES), et se compile également avec -lGL
si on supprime le #define GLFW_INCLUDE_ES2
.
Si nous ajoutons des choses qui ne sont pas dans GLES comme le rendu immédiat glBegin
, le lien échoue comme prévu.
Voir aussi :https://askubuntu.com/questions/244133/how-do-i-get-egl-and-opengles-libraries-for-ubuntu-running-on-virtualbox
Crédits :genpfult a rendu le code beaucoup plus correct.
SDK ARM Mali OpenGL ES
- télécharger depuis :http://malideveloper.arm.com/resources/sdks/opengl-es-sdk-for-linux/
- ouvrir la documentation HTML sur un navigateur
- suivez le "Guide de démarrage rapide", c'est simple
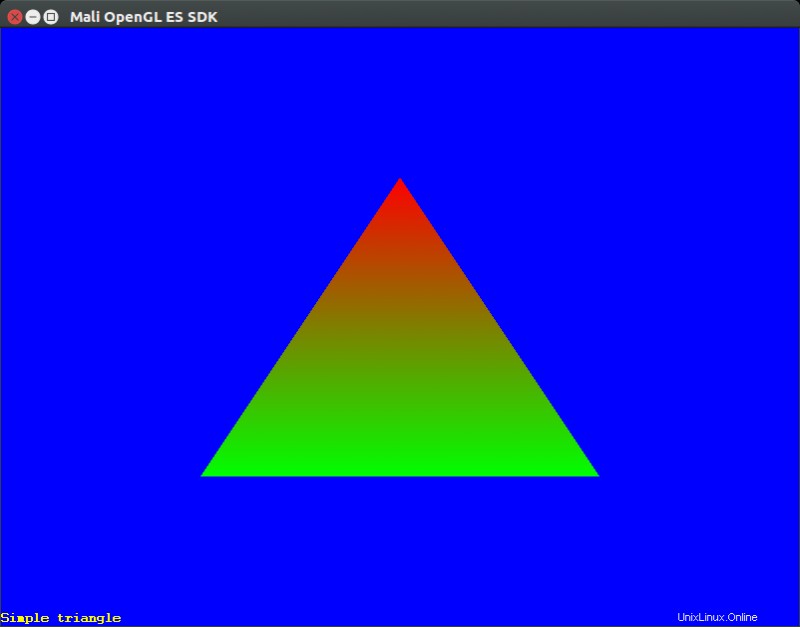
Contient plusieurs exemples open source intéressants + passe-partout du système de fenêtrage (X11 + EGL).
Le système de construction prend en charge la compilation croisée facile pour les SoC ARM / Mali, mais je ne l'ai pas encore testé.
Le composant clé inclus semble être "l'émulateur OpenGL ES" http://malideveloper.arm.com/resources/tools/opengl-es-emulator/ qui "mappe les appels d'API OpenGL ES 3.2 à l'API OpenGL". Mais cela n'est pas livré avec la source, seulement précompilé.
Utilise un CLUF d'entreprise personnalisé qui semble permissif, mais oui, demandez à votre avocat.
Testé sur le SDK v2.4.4.